Program.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
| using System;
using static System.Console;
/// <summary>
/// Mark Hesser
/// February 21, 2018
/// Clue
/// </summary>
namespace Clue
{
class Program
{
//Game and Win counters.
static int Wins = 0, GamesPlayed = 0;
static Game game;
static void Main(string[] args)
{
#region Declarations
bool quit = false;
#endregion
Title = "Clue";
try
{
SetWindowSize(120, 40);
}
catch (ArgumentOutOfRangeException)
{
SetWindowSize(WindowWidth * 2, WindowHeight * 2);
}
//Main Loop
while (!quit)
{
Clear();
Directions();
Init();
while (game.isPlaying)
{
Clear();
if (args.Length > 0)
if (args[0] == "debug")
Debug();
DrawMap();
if (game.GameOver)
{
GameOver();
break;
}
DisplayMessages();
Accuse();
}
Quit(ref quit);
}
}
/// <summary>
/// Initalizes a New Game and resets all variables.
/// </summary>
static void Init()
{
game = new Game();
}
/// <summary>
/// Displays the Directions on the Screen.
/// </summary>
static void Directions()
{
WriteLine(" Welcome to Clue! By Mark Hesser \n");
WriteLine(" One murder… 6 suspects. In this suspenseful Clue game, players have to find out who's " +
"\n responsible for murdering Mr. Boddy of Tudor Mansion in his own home. Get the scoop on the mansion's " +
"\n rooms, weapons and guests and start detecting! Was it Plum with the wrench in the library? Or " +
"\n Green with the candlestick in the study? Eliminate information throughout the game in this classic whodunit. " +
"\n The player who correctly accuses Who, What, and Where wins! \n");
WriteLine(" The first thing you will be asked is to enter your character. This player will be removed from the suspects list. " +
"\n You will be given 8 trys to accuse the correct Suspect, Murder Weapon, and Crime Scene." +
"\n The Gameboard will show all the available people, places, and things to accuse." +
"\n You will be asked to Accuse a Suspect, Weapon, and Room, then the game will calculate a response." +
"\n After you make your accusations, they will be marked on the gameboard with an \"X\"." +
"\n If you make a correct accusation the game will tell you in the Messages area, and mark it's spot with an \"S\". " +
"\n The Game is over when you either run out of Trys, or you successfully solve the mystery.");
WriteLine("\n Good Luck!");
WriteLine();
}
/// <summary>
/// Draws the Map and Available Suspects/Weapons on the Screen
/// </summary>
static void DrawMap()
{
WriteLine();
WriteLine("\t Clue ");
WriteLine("\t________________________________________");
WriteLine("\t| | | | Suspects Weapons");
WriteLine("\t| | | {2} | {0} {1}", game.AS[0], game.AW[0], game.AR[1]);
WriteLine("\t| {2} | | | {0} {1}", game.AS[1], game.AW[1], game.AR[0]);
WriteLine("\t| | |_ | {0} {1}", game.AS[2], game.AW[2]);
WriteLine("\t| | ________________| {0} {1}", game.AS[3], game.AW[3]);
WriteLine("\t| _| | | {0} {1}", game.AS[4], game.AW[4]);
WriteLine("\t|________________ | {2} | {0} {1}", game.AS[5], game.AW[5], game.AR[4]);
WriteLine("\t| _ |_ |");
WriteLine("\t| | ________________| You have {0} Try(s) Remaining", game.NumTrys);
WriteLine("\t| {0} | _ |", game.AR[3]);
WriteLine("\t| | | |");
WriteLine("\t| | | {0} |", game.AR[2]);
WriteLine("\t| | | |");
WriteLine("\t|________________|____|________________|");
}
/// <summary>
/// Prompts the Player to enter a Suspect, CrimeScene
/// </summary>
static void Accuse()
{
WriteLine();
game.Accuse();
}
/// <summary>
/// Display messages to the player.
/// </summary>
static void DisplayMessages()
{
WriteLine();
foreach (Message m in game.Messages)
{
WriteLine("\t" + m.message);
}
WriteLine("\n\t----------------------------------------");
}
/// <summary>
/// Displays the End of the Game
/// </summary>
static void GameOver()
{
WriteLine("\n The Murder was committed by {0} with the {2} in the {1}.",
game.file.Suspect, game.file.Room, game.file.Weapon);
if (game.MurderSolved)
{
WriteLine("\n" + game.m.WIN);
Wins++;
}
else
{
WriteLine("\n" + game.m.LOSE);
}
GamesPlayed++;
WriteLine(" You have won {0} out of {1} Games Played!", Wins, GamesPlayed);
}
/// <summary>
/// Asks the player if they would like to play again.
/// </summary>
/// <param name="quit"></param>
static void Quit(ref bool quit)
{
ConsoleKeyInfo kb;
Write("\n Would you like to play again? (y/n) "); //Put message to the user here.
kb = ReadKey(); //Listens for Key
if (kb.Key == ConsoleKey.N) //Ends the Program
{
quit = true;
}
else if (kb.Key == ConsoleKey.Y)
{
quit = false;
}
else //Tells the User they are stupid and then Quits the program
{
WriteLine("\n You didn't hit Y or N. " +
"\n I'm going to assume you said No. " +
"\n Press Any Key Quit...\a");
ReadKey();
quit = true; //Ends Program
}
}
/// <summary>
/// Debug Menu. Tells you the answer while guessing to test all possible results.
/// </summary>
static void Debug()
{
WriteLine("File: {0} with the {2} in the {1}.",
game.file.Suspect, game.file.Room, game.file.Weapon);
}
}
} |
using System;
using static System.Console;
/// <summary>
/// Mark Hesser
/// February 21, 2018
/// Clue
/// </summary>
namespace Clue
{
class Program
{
//Game and Win counters.
static int Wins = 0, GamesPlayed = 0;
static Game game;
static void Main(string[] args)
{
#region Declarations
bool quit = false;
#endregion
Title = "Clue";
try
{
SetWindowSize(120, 40);
}
catch (ArgumentOutOfRangeException)
{
SetWindowSize(WindowWidth * 2, WindowHeight * 2);
}
//Main Loop
while (!quit)
{
Clear();
Directions();
Init();
while (game.isPlaying)
{
Clear();
if (args.Length > 0)
if (args[0] == "debug")
Debug();
DrawMap();
if (game.GameOver)
{
GameOver();
break;
}
DisplayMessages();
Accuse();
}
Quit(ref quit);
}
}
/// <summary>
/// Initalizes a New Game and resets all variables.
/// </summary>
static void Init()
{
game = new Game();
}
/// <summary>
/// Displays the Directions on the Screen.
/// </summary>
static void Directions()
{
WriteLine(" Welcome to Clue! By Mark Hesser \n");
WriteLine(" One murder… 6 suspects. In this suspenseful Clue game, players have to find out who's " +
"\n responsible for murdering Mr. Boddy of Tudor Mansion in his own home. Get the scoop on the mansion's " +
"\n rooms, weapons and guests and start detecting! Was it Plum with the wrench in the library? Or " +
"\n Green with the candlestick in the study? Eliminate information throughout the game in this classic whodunit. " +
"\n The player who correctly accuses Who, What, and Where wins! \n");
WriteLine(" The first thing you will be asked is to enter your character. This player will be removed from the suspects list. " +
"\n You will be given 8 trys to accuse the correct Suspect, Murder Weapon, and Crime Scene." +
"\n The Gameboard will show all the available people, places, and things to accuse." +
"\n You will be asked to Accuse a Suspect, Weapon, and Room, then the game will calculate a response." +
"\n After you make your accusations, they will be marked on the gameboard with an \"X\"." +
"\n If you make a correct accusation the game will tell you in the Messages area, and mark it's spot with an \"S\". " +
"\n The Game is over when you either run out of Trys, or you successfully solve the mystery.");
WriteLine("\n Good Luck!");
WriteLine();
}
/// <summary>
/// Draws the Map and Available Suspects/Weapons on the Screen
/// </summary>
static void DrawMap()
{
WriteLine();
WriteLine("\t Clue ");
WriteLine("\t________________________________________");
WriteLine("\t| | | | Suspects Weapons");
WriteLine("\t| | | {2} | {0} {1}", game.AS[0], game.AW[0], game.AR[1]);
WriteLine("\t| {2} | | | {0} {1}", game.AS[1], game.AW[1], game.AR[0]);
WriteLine("\t| | |_ | {0} {1}", game.AS[2], game.AW[2]);
WriteLine("\t| | ________________| {0} {1}", game.AS[3], game.AW[3]);
WriteLine("\t| _| | | {0} {1}", game.AS[4], game.AW[4]);
WriteLine("\t|________________ | {2} | {0} {1}", game.AS[5], game.AW[5], game.AR[4]);
WriteLine("\t| _ |_ |");
WriteLine("\t| | ________________| You have {0} Try(s) Remaining", game.NumTrys);
WriteLine("\t| {0} | _ |", game.AR[3]);
WriteLine("\t| | | |");
WriteLine("\t| | | {0} |", game.AR[2]);
WriteLine("\t| | | |");
WriteLine("\t|________________|____|________________|");
}
/// <summary>
/// Prompts the Player to enter a Suspect, CrimeScene
/// </summary>
static void Accuse()
{
WriteLine();
game.Accuse();
}
/// <summary>
/// Display messages to the player.
/// </summary>
static void DisplayMessages()
{
WriteLine();
foreach (Message m in game.Messages)
{
WriteLine("\t" + m.message);
}
WriteLine("\n\t----------------------------------------");
}
/// <summary>
/// Displays the End of the Game
/// </summary>
static void GameOver()
{
WriteLine("\n The Murder was committed by {0} with the {2} in the {1}.",
game.file.Suspect, game.file.Room, game.file.Weapon);
if (game.MurderSolved)
{
WriteLine("\n" + game.m.WIN);
Wins++;
}
else
{
WriteLine("\n" + game.m.LOSE);
}
GamesPlayed++;
WriteLine(" You have won {0} out of {1} Games Played!", Wins, GamesPlayed);
}
/// <summary>
/// Asks the player if they would like to play again.
/// </summary>
/// <param name="quit"></param>
static void Quit(ref bool quit)
{
ConsoleKeyInfo kb;
Write("\n Would you like to play again? (y/n) "); //Put message to the user here.
kb = ReadKey(); //Listens for Key
if (kb.Key == ConsoleKey.N) //Ends the Program
{
quit = true;
}
else if (kb.Key == ConsoleKey.Y)
{
quit = false;
}
else //Tells the User they are stupid and then Quits the program
{
WriteLine("\n You didn't hit Y or N. " +
"\n I'm going to assume you said No. " +
"\n Press Any Key Quit...\a");
ReadKey();
quit = true; //Ends Program
}
}
/// <summary>
/// Debug Menu. Tells you the answer while guessing to test all possible results.
/// </summary>
static void Debug()
{
WriteLine("File: {0} with the {2} in the {1}.",
game.file.Suspect, game.file.Room, game.file.Weapon);
}
}
}
Game.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
361
362
363
364
365
366
367
368
369
| using System;
using System.Collections;
using static System.Console;
namespace Clue
{
public class Game
{
#region Declarations
public Messages m;
public ArrayList Messages;
public Random rand;
public CaseFile file;
public CaseFile clue;
public string Player;
private string player;
public bool isPlaying;
private bool[] bSuspects;
private bool[] bRooms;
private bool[] bWeapons;
public string[] AS;
public string[] AR;
public string[] AW;
public bool Found_Suspect;
public bool Found_CrimeScene;
public bool Found_MurderWeapon;
public bool MurderSolved;
public bool GameOver;
public int NumTrys;
private string[] suspects = Enum.GetNames(typeof(ESuspects));
private string[] rooms = Enum.GetNames(typeof(ERooms));
private string[] weapons = Enum.GetNames(typeof(EWeapons));
#endregion
/// <summary>
/// Default Constructor Method that Initalizes New Game variables when called.
/// In theory, could have multiple Games being played at once. But lets not get ahead of ourselves.
/// </summary>
public Game()
{
isPlaying = true;
m = new Messages();
Messages = new ArrayList();
AddMessage(String.Format("--------------- Messages ---------------"));
rand = new Random();
WriteLine(" Availble Characters.");
foreach (var item in suspects)
{
Write(" {0},", item);
}
Write("\n\n Please enter your Character: ");
ESuspects result;
player = ReadLine();
while (!Enum.TryParse(player, true, out result))
{
WriteLine(" That is not a useable Character... \n");
Write(" Please enter your Character: ");
player = ReadLine();
}
Player = result.ToString();
file = new CaseFile(Player);
clue = new CaseFile(Player, false);
bSuspects = new bool[CaseConst.NUM_SUSPECTS];
for (int i = 0; i < bSuspects.Length; i++)
bSuspects[i] = false;
bRooms = new bool[CaseConst.NUM_ROOMS];
for (int i = 0; i < bRooms.Length; i++)
bRooms[i] = false;
bWeapons = new bool[CaseConst.NUM_WEAPONS];
for (int i = 0; i < bWeapons.Length; i++)
bWeapons[i] = false;
AS = new string[CaseConst.NUM_SUSPECTS];
AR = new string[CaseConst.NUM_ROOMS];
AW = new string[CaseConst.NUM_WEAPONS];
int index = Array.IndexOf(suspects, Player);
bSuspects[index] = true;
ResetAccused();
Found_Suspect = false;
Found_CrimeScene = false;
Found_MurderWeapon = false;
NumTrys = 8;
}
/// <summary>
/// Adds Messages to the Gameboard.
/// </summary>
/// <param name="s"></param>
public void AddMessage(string s)
{
Messages.Add(new Message(s));
}
/// <summary>
/// Resets the Drawing board with an X if the item has been guessed,
/// or an S if the guess was the correct answer.
/// </summary>
public void ResetAccused()
{
for (int i = 0; i < CaseConst.NUM_SUSPECTS; i++)
{
if (bSuspects[i] && suspects[i] == file.Suspect)
{
AS[i] = m.SUSPECT_X[i, 2];
}
else if (bSuspects[i] && suspects[i] != file.Suspect)
{
AS[i] = m.SUSPECT_X[i, 1];
}
else
{
AS[i] = m.SUSPECT_X[i, 0];
}
}
for (int i = 0; i < CaseConst.NUM_ROOMS; i++)
{
if (bRooms[i] && rooms[i] == file.Room)
{
AR[i] = m.ROOMS_X[i, 2];
}
else if (bRooms[i] && rooms[i] != file.Room)
{
AR[i] = m.ROOMS_X[i, 1];
}
else
{
AR[i] = m.ROOMS_X[i, 0];
}
}
for (int i = 0; i < CaseConst.NUM_WEAPONS; i++)
{
if (bWeapons[i] && weapons[i] == file.Weapon)
{
AW[i] = m.WEAPON_X[i, 2];
}
else if (bWeapons[i] && weapons[i] != file.Weapon)
{
AW[i] = m.WEAPON_X[i, 1];
}
else
{
AW[i] = m.WEAPON_X[i, 0];
}
}
}
/// <summary>
/// Prompts the user for their accusation and processes it accordingly.
/// </summary>
public void Accuse()
{
int index;
if (!Found_Suspect)
{
Write(" Accuse a Suspect: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(1, ReadLine(), out clue.Suspect))
{
WriteLine(m.NOT_VALID);
Write("\n Accuse a Suspect: ");
}
index = Array.IndexOf(suspects, clue.Suspect);
bSuspects[index] = true;
//Checks to see if accusation is right.
Found_Suspect = Compare(1, clue.Suspect);
}
if (!Found_MurderWeapon)
{
Write(" What Weapon was used: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(3, ReadLine(), out clue.Weapon))
{
WriteLine(m.NOT_VALID);
Write("\n What Weapon was used: ");
}
index = Array.IndexOf(weapons, clue.Weapon);
bWeapons[index] = true;
//Checks to see if accusation is right.
Found_MurderWeapon = Compare(3, clue.Weapon);
}
if (!Found_CrimeScene)
{
Write(" Where is the Crime Scene: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(2, ReadLine(), out clue.Room))
{
WriteLine(m.NOT_VALID);
Write("\n Where is the Crime Scene: ");
}
index = Array.IndexOf(rooms, clue.Room);
bRooms[index] = true;
//Checks to see if accusation is right.
Found_CrimeScene = Compare(2, clue.Room);
}
//Don't know why I put this here, but it works so it stays.
NumTrys--;
if (!MurderSolved && !GameOver)
{
MurderSolved = CheckGame();
}
ResetAccused();
}
/// <summary>
/// Check 1 for suspect, Check 2 for room, Check 3 for check weapon,
/// This Method Checks to see if the input value is valid and returns a boolean.
/// Use to redirect a string input to an output.
/// </summary>
/// <param name="check"></param>
/// <returns></returns>
private bool isValid(int check, string value, out string result)
{
bool valid = false;
result = value;
switch (check)
{
case 1: //Suspect
{
if (Enum.TryParse(value, true, out ESuspects compare))
{
result = compare.ToString();
valid = true;
}
break;
}
case 2: //Room
{
if (Enum.TryParse(value, true, out ERooms compare))
{
result = compare.ToString();
valid = true;
}
break;
}
case 3: //Weapon
{
if (Enum.TryParse(value, true, out EWeapons compare))
{
result = compare.ToString();
valid = true;
}
break;
}
default:
{
result = value;
break;
}
}
return valid;
}
/// <summary>
/// Check 1 for suspect, Check 2 for room, Check 3 for check weapon,
/// </summary>
/// <param name="check"></param>
/// <param name="value"></param>
/// <returns></returns>
private bool Compare(int check, string value)
{
bool match = false;
switch (check)
{
case 1:
{
if (Enum.TryParse(value, true, out ESuspects compare))
{
if (compare.ToString() == file.Suspect)
{
match = true;
AddMessage(String.Format(m.SUSPECT_FOUND, file.Suspect));
}
}
break;
}
case 2:
{
if (Enum.TryParse(value, true, out ERooms compare))
{
if (compare.ToString() == file.Room)
{
match = true;
AddMessage(String.Format(m.ROOM_FOUND, file.Room));
}
}
break;
}
case 3:
{
if (Enum.TryParse(value, true, out EWeapons compare))
{
if (compare.ToString() == file.Weapon)
{
match = true;
AddMessage(String.Format(m.WEAPON_FOUND, file.Weapon));
}
}
break;
}
default:
break;
}
return match;
}
/// <summary>
/// Checks to see if the player has won or not.
/// If Number of Trys is 0, triggers game over.
/// </summary>
/// <returns></returns>
private bool CheckGame()
{
bool solved = false;
if (Found_Suspect && Found_CrimeScene && Found_MurderWeapon)
{
solved = true;
GameOver = true;
}
else if (NumTrys == 0)
GameOver = true;
return solved;
}
//I stopped caring about commenting around here, because this project is so complicated, I don't even understand how it works anymore.
//But it works, so I'm going to leave it alone.
}
} |
using System;
using System.Collections;
using static System.Console;
namespace Clue
{
public class Game
{
#region Declarations
public Messages m;
public ArrayList Messages;
public Random rand;
public CaseFile file;
public CaseFile clue;
public string Player;
private string player;
public bool isPlaying;
private bool[] bSuspects;
private bool[] bRooms;
private bool[] bWeapons;
public string[] AS;
public string[] AR;
public string[] AW;
public bool Found_Suspect;
public bool Found_CrimeScene;
public bool Found_MurderWeapon;
public bool MurderSolved;
public bool GameOver;
public int NumTrys;
private string[] suspects = Enum.GetNames(typeof(ESuspects));
private string[] rooms = Enum.GetNames(typeof(ERooms));
private string[] weapons = Enum.GetNames(typeof(EWeapons));
#endregion
/// <summary>
/// Default Constructor Method that Initalizes New Game variables when called.
/// In theory, could have multiple Games being played at once. But lets not get ahead of ourselves.
/// </summary>
public Game()
{
isPlaying = true;
m = new Messages();
Messages = new ArrayList();
AddMessage(String.Format("--------------- Messages ---------------"));
rand = new Random();
WriteLine(" Availble Characters.");
foreach (var item in suspects)
{
Write(" {0},", item);
}
Write("\n\n Please enter your Character: ");
ESuspects result;
player = ReadLine();
while (!Enum.TryParse(player, true, out result))
{
WriteLine(" That is not a useable Character... \n");
Write(" Please enter your Character: ");
player = ReadLine();
}
Player = result.ToString();
file = new CaseFile(Player);
clue = new CaseFile(Player, false);
bSuspects = new bool[CaseConst.NUM_SUSPECTS];
for (int i = 0; i < bSuspects.Length; i++)
bSuspects[i] = false;
bRooms = new bool[CaseConst.NUM_ROOMS];
for (int i = 0; i < bRooms.Length; i++)
bRooms[i] = false;
bWeapons = new bool[CaseConst.NUM_WEAPONS];
for (int i = 0; i < bWeapons.Length; i++)
bWeapons[i] = false;
AS = new string[CaseConst.NUM_SUSPECTS];
AR = new string[CaseConst.NUM_ROOMS];
AW = new string[CaseConst.NUM_WEAPONS];
int index = Array.IndexOf(suspects, Player);
bSuspects[index] = true;
ResetAccused();
Found_Suspect = false;
Found_CrimeScene = false;
Found_MurderWeapon = false;
NumTrys = 8;
}
/// <summary>
/// Adds Messages to the Gameboard.
/// </summary>
/// <param name="s"></param>
public void AddMessage(string s)
{
Messages.Add(new Message(s));
}
/// <summary>
/// Resets the Drawing board with an X if the item has been guessed,
/// or an S if the guess was the correct answer.
/// </summary>
public void ResetAccused()
{
for (int i = 0; i < CaseConst.NUM_SUSPECTS; i++)
{
if (bSuspects[i] && suspects[i] == file.Suspect)
{
AS[i] = m.SUSPECT_X[i, 2];
}
else if (bSuspects[i] && suspects[i] != file.Suspect)
{
AS[i] = m.SUSPECT_X[i, 1];
}
else
{
AS[i] = m.SUSPECT_X[i, 0];
}
}
for (int i = 0; i < CaseConst.NUM_ROOMS; i++)
{
if (bRooms[i] && rooms[i] == file.Room)
{
AR[i] = m.ROOMS_X[i, 2];
}
else if (bRooms[i] && rooms[i] != file.Room)
{
AR[i] = m.ROOMS_X[i, 1];
}
else
{
AR[i] = m.ROOMS_X[i, 0];
}
}
for (int i = 0; i < CaseConst.NUM_WEAPONS; i++)
{
if (bWeapons[i] && weapons[i] == file.Weapon)
{
AW[i] = m.WEAPON_X[i, 2];
}
else if (bWeapons[i] && weapons[i] != file.Weapon)
{
AW[i] = m.WEAPON_X[i, 1];
}
else
{
AW[i] = m.WEAPON_X[i, 0];
}
}
}
/// <summary>
/// Prompts the user for their accusation and processes it accordingly.
/// </summary>
public void Accuse()
{
int index;
if (!Found_Suspect)
{
Write(" Accuse a Suspect: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(1, ReadLine(), out clue.Suspect))
{
WriteLine(m.NOT_VALID);
Write("\n Accuse a Suspect: ");
}
index = Array.IndexOf(suspects, clue.Suspect);
bSuspects[index] = true;
//Checks to see if accusation is right.
Found_Suspect = Compare(1, clue.Suspect);
}
if (!Found_MurderWeapon)
{
Write(" What Weapon was used: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(3, ReadLine(), out clue.Weapon))
{
WriteLine(m.NOT_VALID);
Write("\n What Weapon was used: ");
}
index = Array.IndexOf(weapons, clue.Weapon);
bWeapons[index] = true;
//Checks to see if accusation is right.
Found_MurderWeapon = Compare(3, clue.Weapon);
}
if (!Found_CrimeScene)
{
Write(" Where is the Crime Scene: ");
//Works Like TryParse, but for checking if input can be compared to file.
while (!isValid(2, ReadLine(), out clue.Room))
{
WriteLine(m.NOT_VALID);
Write("\n Where is the Crime Scene: ");
}
index = Array.IndexOf(rooms, clue.Room);
bRooms[index] = true;
//Checks to see if accusation is right.
Found_CrimeScene = Compare(2, clue.Room);
}
//Don't know why I put this here, but it works so it stays.
NumTrys--;
if (!MurderSolved && !GameOver)
{
MurderSolved = CheckGame();
}
ResetAccused();
}
/// <summary>
/// Check 1 for suspect, Check 2 for room, Check 3 for check weapon,
/// This Method Checks to see if the input value is valid and returns a boolean.
/// Use to redirect a string input to an output.
/// </summary>
/// <param name="check"></param>
/// <returns></returns>
private bool isValid(int check, string value, out string result)
{
bool valid = false;
result = value;
switch (check)
{
case 1: //Suspect
{
if (Enum.TryParse(value, true, out ESuspects compare))
{
result = compare.ToString();
valid = true;
}
break;
}
case 2: //Room
{
if (Enum.TryParse(value, true, out ERooms compare))
{
result = compare.ToString();
valid = true;
}
break;
}
case 3: //Weapon
{
if (Enum.TryParse(value, true, out EWeapons compare))
{
result = compare.ToString();
valid = true;
}
break;
}
default:
{
result = value;
break;
}
}
return valid;
}
/// <summary>
/// Check 1 for suspect, Check 2 for room, Check 3 for check weapon,
/// </summary>
/// <param name="check"></param>
/// <param name="value"></param>
/// <returns></returns>
private bool Compare(int check, string value)
{
bool match = false;
switch (check)
{
case 1:
{
if (Enum.TryParse(value, true, out ESuspects compare))
{
if (compare.ToString() == file.Suspect)
{
match = true;
AddMessage(String.Format(m.SUSPECT_FOUND, file.Suspect));
}
}
break;
}
case 2:
{
if (Enum.TryParse(value, true, out ERooms compare))
{
if (compare.ToString() == file.Room)
{
match = true;
AddMessage(String.Format(m.ROOM_FOUND, file.Room));
}
}
break;
}
case 3:
{
if (Enum.TryParse(value, true, out EWeapons compare))
{
if (compare.ToString() == file.Weapon)
{
match = true;
AddMessage(String.Format(m.WEAPON_FOUND, file.Weapon));
}
}
break;
}
default:
break;
}
return match;
}
/// <summary>
/// Checks to see if the player has won or not.
/// If Number of Trys is 0, triggers game over.
/// </summary>
/// <returns></returns>
private bool CheckGame()
{
bool solved = false;
if (Found_Suspect && Found_CrimeScene && Found_MurderWeapon)
{
solved = true;
GameOver = true;
}
else if (NumTrys == 0)
GameOver = true;
return solved;
}
//I stopped caring about commenting around here, because this project is so complicated, I don't even understand how it works anymore.
//But it works, so I'm going to leave it alone.
}
}
CaseFile.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
| namespace Clue
{
public enum ESuspects
{
Plum = 1, White, Scarlet, Green, Mustard, Peacock
}
public enum ERooms
{
Kitchen = 1, Lounge, Study, Library, Hall
}
public enum EWeapons
{
Rope = 1, Knife, Wrench, Revolver, Candlestick, Poisen
}
public class CaseConst
{
public const int NUM_SUSPECTS = 6;
public const int NUM_ROOMS = 5;
public const int NUM_WEAPONS = 6;
}
public class CaseFile
{
private Suspects suspect;
public string Suspect;
private Weapons weapon;
public string Weapon;
private Rooms room;
public string Room;
public CaseFile(string player, bool random = true)
{
if (random == true)
{
suspect = new Suspects(player);
room = new Rooms();
weapon = new Weapons();
Room = room.CrimeScene;
Weapon = weapon.MurderWeapon;
Suspect = suspect.MurderSuspect;
}
}
public CaseFile(string suspect, string room, string weapon)
{
Room = room;
Weapon = weapon;
Suspect = suspect;
}
}
} |
namespace Clue
{
public enum ESuspects
{
Plum = 1, White, Scarlet, Green, Mustard, Peacock
}
public enum ERooms
{
Kitchen = 1, Lounge, Study, Library, Hall
}
public enum EWeapons
{
Rope = 1, Knife, Wrench, Revolver, Candlestick, Poisen
}
public class CaseConst
{
public const int NUM_SUSPECTS = 6;
public const int NUM_ROOMS = 5;
public const int NUM_WEAPONS = 6;
}
public class CaseFile
{
private Suspects suspect;
public string Suspect;
private Weapons weapon;
public string Weapon;
private Rooms room;
public string Room;
public CaseFile(string player, bool random = true)
{
if (random == true)
{
suspect = new Suspects(player);
room = new Rooms();
weapon = new Weapons();
Room = room.CrimeScene;
Weapon = weapon.MurderWeapon;
Suspect = suspect.MurderSuspect;
}
}
public CaseFile(string suspect, string room, string weapon)
{
Room = room;
Weapon = weapon;
Suspect = suspect;
}
}
}
Messages.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
| namespace Clue
{
public class Messages
{
public string WIN = " You solved the Mystery. You Win!";
public string LOSE = " The suspect got away... You Lose!";
public string SUSPECT_FOUND = "* {0} Commited the Murder!";
public string ROOM_FOUND = "* The Murder was commited in the {0}!";
public string WEAPON_FOUND = "* The Murder was commited with the {0}!";
public string[,] SUSPECT_X = new string[,]
{
{ "[ ] - Plum ", "[X] - Plum ", "[S] - Plum " },
{ "[ ] - White ", "[X] - White ", "[S] - White " },
{ "[ ] - Scarlet", "[X] - Scarlet", "[S] - Scarlet" },
{ "[ ] - Green ", "[X] - Green ", "[S] - Green " },
{ "[ ] - Mustard", "[X] - Mustard", "[S] - Mustard" },
{ "[ ] - Peacock", "[X] - Peacock", "[S] - Peacock" }
};
public string[,] WEAPON_X = new string[,]
{
{"[ ] - Rope ", "[X] - Rope ", "[S] - Rope "},
{"[ ] - Knife ", "[X] - Knife ", "[S] - Knife "},
{"[ ] - Wrench ", "[X] - Wrench ", "[S] - Wrench "},
{"[ ] - Revolver ", "[X] - Revolver ", "[S] - Revolver "},
{"[ ] - Candlestick", "[X] - Candlestick", "[S] - Candlestick"},
{"[ ] - Poisen ", "[X] - Poisen ", "[S] - Poisen "}
};
public string[,] ROOMS_X = new string[,]
{
{"[ ] - Kitchen", "[X] - Kitchen", "[S] - Kitchen" },
{"[ ] - Lounge", "[X] - Lounge", "[S] - Lounge"},
{"[ ] - Study", "[X] - Study", "[S] - Study"},
{"[ ] - Library", "[X] - Library", "[S] - Library"},
{"[ ] - Hall", "[X] - Hall", "[S] - Hall"},
};
public string NOT_VALID = " That is not a valid option... Please Choose from the List above";
}
public class Message
{
public string message;
public Message(string m)
{
message = m;
}
}
} |
namespace Clue
{
public class Messages
{
public string WIN = " You solved the Mystery. You Win!";
public string LOSE = " The suspect got away... You Lose!";
public string SUSPECT_FOUND = "* {0} Commited the Murder!";
public string ROOM_FOUND = "* The Murder was commited in the {0}!";
public string WEAPON_FOUND = "* The Murder was commited with the {0}!";
public string[,] SUSPECT_X = new string[,]
{
{ "[ ] - Plum ", "[X] - Plum ", "[S] - Plum " },
{ "[ ] - White ", "[X] - White ", "[S] - White " },
{ "[ ] - Scarlet", "[X] - Scarlet", "[S] - Scarlet" },
{ "[ ] - Green ", "[X] - Green ", "[S] - Green " },
{ "[ ] - Mustard", "[X] - Mustard", "[S] - Mustard" },
{ "[ ] - Peacock", "[X] - Peacock", "[S] - Peacock" }
};
public string[,] WEAPON_X = new string[,]
{
{"[ ] - Rope ", "[X] - Rope ", "[S] - Rope "},
{"[ ] - Knife ", "[X] - Knife ", "[S] - Knife "},
{"[ ] - Wrench ", "[X] - Wrench ", "[S] - Wrench "},
{"[ ] - Revolver ", "[X] - Revolver ", "[S] - Revolver "},
{"[ ] - Candlestick", "[X] - Candlestick", "[S] - Candlestick"},
{"[ ] - Poisen ", "[X] - Poisen ", "[S] - Poisen "}
};
public string[,] ROOMS_X = new string[,]
{
{"[ ] - Kitchen", "[X] - Kitchen", "[S] - Kitchen" },
{"[ ] - Lounge", "[X] - Lounge", "[S] - Lounge"},
{"[ ] - Study", "[X] - Study", "[S] - Study"},
{"[ ] - Library", "[X] - Library", "[S] - Library"},
{"[ ] - Hall", "[X] - Hall", "[S] - Hall"},
};
public string NOT_VALID = " That is not a valid option... Please Choose from the List above";
}
public class Message
{
public string message;
public Message(string m)
{
message = m;
}
}
}
Suspects.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
| using System;
namespace Clue
{
public class Suspects
{
public Suspects(string player)
{
do
{
MurderSuspect = ((ESuspects)suspects.GetValue(RandNum())).ToString();
} while (MurderSuspect.ToUpper() == player.ToUpper());
}
private Array suspects = Enum.GetValues(typeof(ESuspects));
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_SUSPECTS);
}
public string MurderSuspect;
}
} |
using System;
namespace Clue
{
public class Suspects
{
public Suspects(string player)
{
do
{
MurderSuspect = ((ESuspects)suspects.GetValue(RandNum())).ToString();
} while (MurderSuspect.ToUpper() == player.ToUpper());
}
private Array suspects = Enum.GetValues(typeof(ESuspects));
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_SUSPECTS);
}
public string MurderSuspect;
}
}
Rooms.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| using System;
namespace Clue
{
public class Rooms
{
public Rooms()
{
CrimeScene = ((ERooms)rooms.GetValue(RandNum())).ToString();
}
private Array rooms = Enum.GetValues(typeof(ERooms));
public string CrimeScene;
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_ROOMS);
}
}
} |
using System;
namespace Clue
{
public class Rooms
{
public Rooms()
{
CrimeScene = ((ERooms)rooms.GetValue(RandNum())).ToString();
}
private Array rooms = Enum.GetValues(typeof(ERooms));
public string CrimeScene;
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_ROOMS);
}
}
}
Weapons.cs
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| using System;
namespace Clue
{
public class Weapons
{
public Weapons()
{
MurderWeapon = ((EWeapons)weapons.GetValue(RandNum())).ToString();
}
private Array weapons = Enum.GetValues(typeof(EWeapons));
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_WEAPONS);
}
public string MurderWeapon;
}
} |
using System;
namespace Clue
{
public class Weapons
{
public Weapons()
{
MurderWeapon = ((EWeapons)weapons.GetValue(RandNum())).ToString();
}
private Array weapons = Enum.GetValues(typeof(EWeapons));
private Random rand = new Random();
private int RandNum()
{
return rand.Next(CaseConst.NUM_WEAPONS);
}
public string MurderWeapon;
}
}
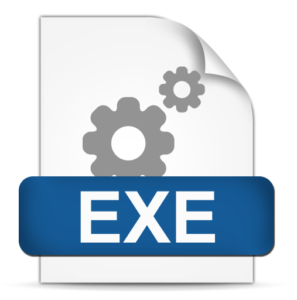