using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
/// <summary>
/// #################
/// # Mark Hesser #
/// # Dec 20, 2017 #
/// # WebAddress #
/// #############################################################################################
/// # Write a program named WebAddress that asks a user for a business name. Suggest a #
/// # good Web address by adding www. to the front of the name, removing all the spaces from #
/// # the name, and adding .com to the end of the na,e. For example, a good Web address #
/// # for Acme Plumbing and Supply is www.AcmePlumbingandSupply.com. #
/// #############################################################################################
/// </summary>
namespace WebAddress
{
class WebAddress
{
static void Main(string[] args)
{
#region Declarations
string[] reserved = new string[10] { ";", "/", "?", ":", "@", "&", "=", "+", "$", "," };
string[] unreserved = new string[9] { "-", "_", ".", "!", "~", "*", "’", "(", ")" };
string[] unwise = new string[8] { "{", "}", "|", "\\", "^", "[", "]", "\’" };
string[] delims = new string[5] { "<", ">", "#", "%", "\"" };
string _www = "www.", _com = ".com", name, url;
#endregion
//Title
Clear(); Title = "Web Address";
//Opening Statement
WriteLine("This program suggests good web urls from your Business Name");
Write("Please Enter Your Business Name: ");
//Input Business Name
name = ReadLine();
#region Calculations
//Remove Illegal characters from name
name = name.Replace(" ", string.Empty);
for (int i = 0; i < reserved.Length; i++)
name = name.Replace(reserved[i], string.Empty);
for (int i = 0; i < unreserved.Length; i++)
name = name.Replace(unreserved[i], string.Empty);
for (int i = 0; i < unwise.Length; i++)
name = name.Replace(unwise[i], string.Empty);
for (int i = 0; i < delims.Length; i++)
name = name.Replace(delims[i], string.Empty);
#endregion
//Append www.name.com
url = _www + name + _com;
WriteLine("Your URL suggestion is \"{0}\"", url);
ReadKey();
}
}
}
[/code]
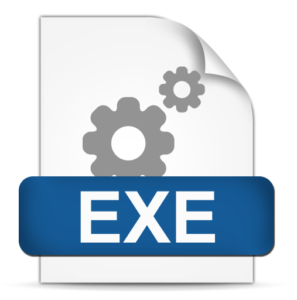
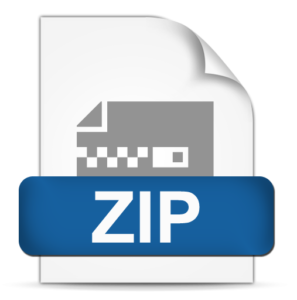