# Dec 10, 17
# Eggs */
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
namespace Eggs
{
class Program
{
static void Main(string[] args)
{
//Declarations
bool programSelectError = false, Menu = true;
int programSelect;
ConsoleKeyInfo kb;
while (Menu)
{
Clear(); Title = "Main Menu";
//Main Menu
WriteLine("Programs:");
WriteLine(" 1. Eggs \n 2. Eggs Interactive \n\n Q. Quit");
if (programSelectError) //Invalid Selection
{
WriteLine("\nThat is not a Valid Choice, Enter # 1-2 or Q to Quit");
programSelectError = false;
}
Write("\nPlease Select the Program you would like to run: ");
kb = ReadKey(); //Listens for Key
if (kb.Key == ConsoleKey.Q)
{
Menu = false;
}
string programKey = kb.KeyChar.ToString();
Int32.TryParse(programKey, out programSelect);
switch (programSelect) //Program Selection
{
case 1: Menu = false; NonInteractive(); break;
case 2: Menu = false; Interactive(); break;
default: programSelectError = true; break;
}
}
}
static void NonInteractive()
{
Clear(); Title = "Eggs";
WriteLine("This program converts total eggs laid into dozens\n");
//Declarations
int[] chicken = new int[4] { 31, 27, 16, 19 };
int eggSum = 0, eggDozens, eggRemainder;
const byte dozens = 12;
//Calcuations
for (int i = 0; i < chicken.Length; i++)
{
WriteLine(" Chicken {0} laid {1} eggs\n",(i+1), chicken[i]);
eggSum += chicken[i];
}
eggDozens = eggSum / dozens;
eggRemainder = eggSum % dozens;
//Output
WriteLine("A total of {0} eggs is {1} dozen and {2} eggs.\n", eggSum, eggDozens, eggRemainder);
WriteLine("Press any key to Exit"); ReadKey();
}
static void Interactive()
{
Clear(); Title = "Eggs Interactive";
//Declarations
int numChickens, eggSum = 0, eggDozens, eggRemainder;
const byte dozens = 12;
//Opening Statement
WriteLine("This program converts total eggs laid into dozens.\n");
Write("How many chickens do you have: ");
while (!Int32.TryParse(ReadLine(), out numChickens))
{
WriteLine("That is not a number…\n");
Write("How many chickens do you have: ");
}
//Declare Chicken Array
int[] chicken = new int[numChickens];
//Input
for (int i = 0; i < chicken.Length; i++)
{
Write("\nHow many eggs did Chicken {0} lay: ", (i + 1));
while (!Int32.TryParse(ReadLine(), out chicken[i]))
{
WriteLine("That is not a number…\n");
Write("How many eggs did Chicken {0} lay: ", (i + 1));
}
//Add to sum
eggSum += chicken[i];
}
//Calculations
eggDozens = eggSum / dozens;
eggRemainder = eggSum % dozens;
//Output
WriteLine();
if (eggRemainder == 0)
{
WriteLine("Your chickens laid a total of {0} eggs " +
"which is {1} dozen.\n", eggSum, eggDozens);
}
else
{
WriteLine("Your chickens laid a total of {0} eggs " +
"which is {1} dozen and {2} eggs.\n", eggSum, eggDozens, eggRemainder);
}
WriteLine("Press any key to Exit"); ReadKey();
}
}
}
[/code]
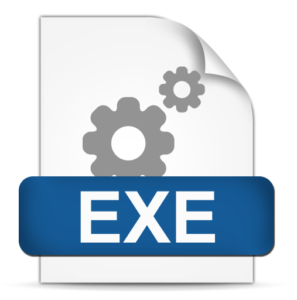
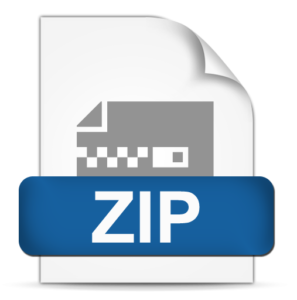