using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
/// <summary>
/// #################################
/// # Mark Hesser #
/// # Dec 20, 2017 #
/// # DisplayMultiplicationTable #
/// #########################################################################################
/// # Write an application named DisplayMultiplicationTable that displays a table of the #
/// # products of every combination of two integers from 1 through 10 #
/// #########################################################################################
/// </summary>
namespace DisplayMultiplicationTable
{
class TimesTable
{
static void Main(string[] args)
{
#region Declarations
int[,] table = new int[11, 11];
string X = "X";
#endregion
Clear(); Title = "Multiplication Table";
SetWindowSize(47, 15);
//Opening Statement
WriteLine("\n Multiplication Table for 1 through 10\n");
#region Setup Arrays
//Set up 1st spot in the array
table[0, 0] = 0;
//Fill First Row and Column of Array
for (int i = 1; i < table.GetLength(0); ++i)
{
table[0, i] = i;
table[i, 0] = i;
}
// Fill 2D Array
for (int row = 1; row < table.GetLength(0); ++row)
{
for (int column = 1; column < table.GetLength(1); ++column)
{
table[row, column] = (row) * (column); //Multiplying 1st Row by 1st Column to get result
}
}
#endregion
#region Display Table
//Display 2D Array
for (int r = 0; r < table.GetLength(0); ++r)
{
for (int c = 0; c < table.GetLength(1); ++c)
{
if (r == 0 && c == 0) Write("{0,4}", X);
else Write("{0,4}", table[r, c]);
}
WriteLine();
}
#endregion
ReadKey();
}
}
}
[/code]
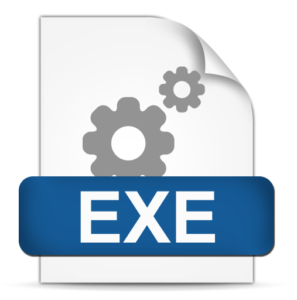
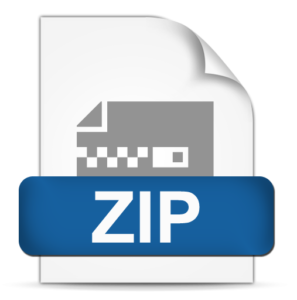