using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static System.Console;
/// <summary>
/// #################
/// # Mark Hesser #
/// # Dec 20, 2017 #
/// # DailyTemps #
/// #####################################################################################################
/// # Write an application named DailyTemps that continuously prompts a user for a series #
/// # of daily high temperatures until the user enters a sentinel value. Valid temperatures #
/// # range from -20 through 130 Fahrenheit. When the user enters a valid temperature, #
/// # add it to a total; when the user enters an invalid temperature, display an error message. #
/// # Before the program ends, display the number of temperatures entered and the average temperatures. #
/// #####################################################################################################
/// </summary>
namespace DailyTemps
{
class DailyTemps
{
static void Main(string[] args)
{
#region Declarations
double temp = 0, sum = 0, average = 0;
int i = 1;
bool loop = true;
List<double> temperatures = new List<double>();
#endregion
//Opening Statement
Clear(); Title = "Daily Temps";
WriteLine("This program records daily high temperatures " +
"and then calculates the average temperature. \nEnter 999 is to end input.");
//Prompt for temperature
WriteLine("\nPlease enter a number between -20 and 130");
#region Main Input Loop
while (loop)
{
Write("Temp {0}: ", i);
//Input Data
while (!Double.TryParse(ReadLine(), out temp))
{
WriteLine("\aThat is not a number… \nPlease enter a number between – 20 and 130");
Write("Temp {0}: ", i);
}
// If 999 is entered
if (temp == 999)
{
WriteLine("\nEscape Number entered… 999 will not be added");
loop = false;
}
//Error Checking
else if (temp < -20 || temp > 130)
{
WriteLine("\aInvalid Range… \nPlease enter a number between – 20 and 130");
}
else
{
temperatures.Add(temp);
i++;
}
}
#endregion
#region Calculations
sum = temperatures.Sum();
average = sum / temperatures.Count();
average = Math.Round(average, 2);
#endregion
//Output Average
WriteLine("\nTotal Temp Entries: {0}", temperatures.Count());
WriteLine("Average Temperature: {0}", average);
WriteLine("\nPress Any Key to Quit"); ReadKey();
}
}
}
[/code]
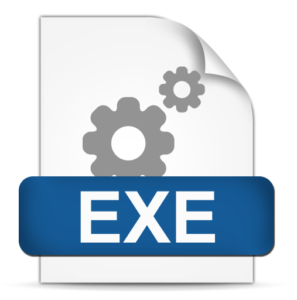
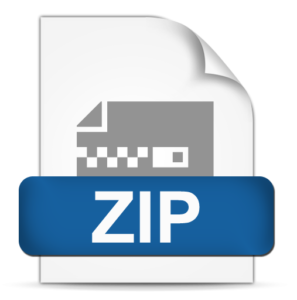